Html Test Runner Python
Testing determines whether software runs correctly based on specific inputsand identifies defects that need to be fixed.
This tool allows you to run any Python demo code online and helps you to test any python code from your browser without any configuration. This tool provides you any Python version from Python 2.7, Python 3.2, Python 3.3, Python 3.4, Python 3.5, Python 3.6, Python 3.7, Python 3.8 and runs your Python code in our sandbox environment. The test contains 40 questions and there is no time limit. The test is not official, it's just a nice way to see how much you know, or don't know, about HTML. Count Your Score. You will get 1 point for each correct answer. At the end of the Quiz, your total score will be displayed. Maximum score is 40 points.
Why is testing important?
As software scales in codebase size, it's impossible for a person or evena large team to keep up with all of the changes andthe interactions between the changes. Automated testing is the only provenmethod for building reliable software once they grow past the point of asimple prototype. Many major software program development failures can betraced back to inadequate or a complete lack of testing.
It's impossible to know whether software works properly unless it is tested.While testing can be done manually, by a user clicking buttons or typing ininput, it should be performed automatically by writing software programs thattest the application under test.
There are many forms of testing and they should all be used together. Whena single function of a program is isolated for testing, that is calledunit testing. Testing more than a single functionin an application at the same time is known asintegration testing.User interface testing ensures the correctness of how a user wouldinteract with the software. There are even more forms of testing that largeprograms need, such as load testing, database testing, andbrowser testing (for web applications).
Testing in Python
Python software development culture is heavy on software testing. BecausePython is a dynamically-typed language as opposed to a statically-typedlanguage, testing takes on even greater importance for ensuring programcorrectness.
Python testing tools
The Python ecosystem has a wealth of tools to make it easier to runyour tests and interpret the results. The following tools encompasstest runners, coverage reports and related libraries.

green is a test runner that haspretty printing on output to make the results easier to read andunderstand.
requestium merges theRequests library with Selenium to make it easier to run automatedbrowser tests.
coverage.py is a tool formeasuring code coverage when running tests.
Testing resources
The Minimum Viable Test Suiteshows how to set unit tests and integration tests for a Flask exampleapplication.
BDD Testing a Restful Web Application in Pythonis an introduction to behavior-driven development (BDD) and usesa Flask web application as an example project forlearning.
Testing, for people who hate testingprovides examples for how to improve your testing environment suchas using a new test harness and getting your test suite to run fast.
Getting started with Pytestgoes over some code challenges as examples for how to use Pytest inyour own projects.
Good test, bad testexplains the difference between a 'good' test case and one that is notas useful. Along the way the post breaks down some myths about commontesting subjects such as code coverage, assertions and mocking.
Python Testing is a site devoted to testingin - you guessed it - the Python programming language.
In praise of property-based testingis an article by the author of the Hypothesistesting tool written in Python. The article explains the shortcomings ofexample-based tests and how property-based testing can augment or replacethose example-based tests to find more defects in software. There is alsoa greatintroductory post on Hypothesisthat goes into further detail about getting your first Hypothesis testsup and running.
Google has a testing blog wherethey write about various aspects of testing software at scale.
A beginner's guide to Python testingcovers test-driven development for unit, integration and smoke tests.
Testing a Twilio Interactive Voice Response (IVR) System With Python and pytestis an incredibly in-depth tutorial on testing a Python-powered IVRusing pytest. There is also a tutorial onhow to build the IVR before this testing tutorial,although you can just clone it to jump into the testing walkthrough.
Still confused about the difference between unit, functional andintegration tests? Check out thistop answer on Stack Overflowto that very question.
Testing Python applications with Pytestwalks through the basics of using Pytest and some more advancedways to use it such as continuous testing through Semiphore CI.
The cleaning hand of Pytestprovides a couple of case studies for how companies set up their testingsystems. It then gives the boilerplate code the author uses for Pytestand goes through a bunch of code samples for common situations.
Using pytest with Djangoshows how to get a basic pytest testcase running for a Django project and explains why the author preferspytest over standard unittest testing.
Distributed Testing with Selenium Grid and Dockershows how to distribute automated, browser tests with Selenium Grid andDocker Swarm. It also looks at how to run tests against a number ofbrowsers and automate the provisioning and deprovisioning of machines tokeep costs down.
Principles of Automated Testingexplains how to prioritize what to test, goes through some levels oftesting from unit tointegration and examines when to use exampleand bulk tests.
How to run tests continuously while codingcontains a Python script that uses thewatchdog to check for changesto source code files in your project directory. If changes are detectedthen your tests will be run to check that everything is still workingas intended.
8 great pytest pluginsis a list of plugins that go with PyTest and help with tasks like reducingthe friction around testing Django, as well as runningtests in parallel.
This test-driven development series shows you how to write an interpreterin Python and contains a ton of great code samples to learn from:
Pytest leakingexamines situations where tests leak memory and can cause abnormalresults if they are not fixed.
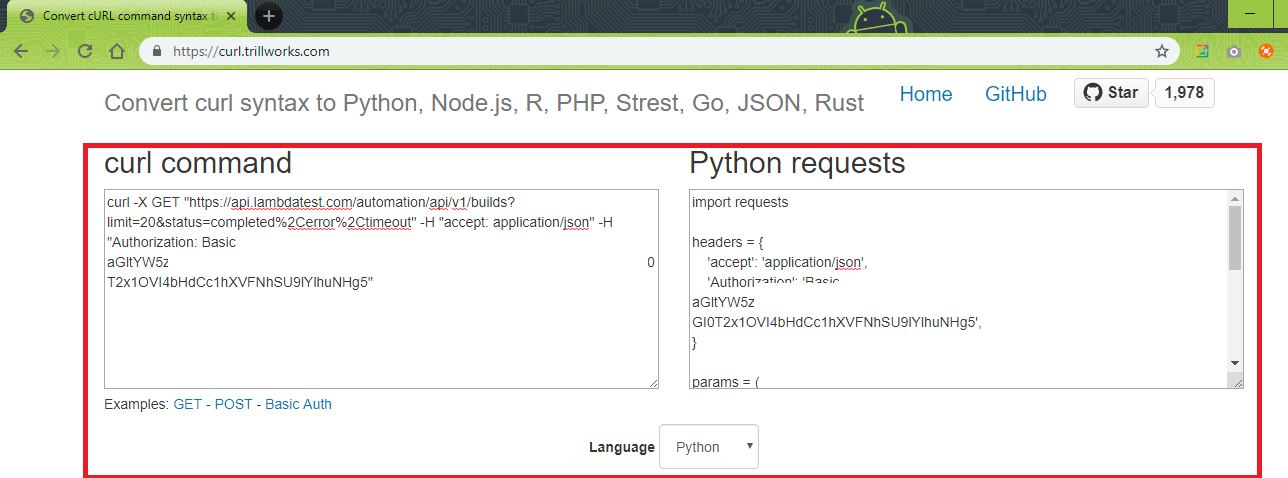
Mocking resources
Mocking allows you to isolate parts of your code under test and avoidareas that are not critical to the tests you are writing. For example,you may want to test how you handle text message responses but do notwant to actually receive text messages in your application. You canmock a part of the code that would provide a happy-path scenario soyou can run your tests properly. The following resources show you how touse mocks in your test cases.
Getting Started with Mocking in Pythonprovides a whole code example based on a blog project that showshow to use
mock
when testing.Python Mocking 101: Fake It Before You Make Itexplains what mocking is and is not, and shows how to use the
patch
function to accomplish it in your project.Revisiting Unit Testing and Mocking in Pythonis a follow-up post that expands upon using thepatch
functionalong with dependency injection.Mocks and Monkeypatching in Pythonuses the
mock
andmonkeypatch
tools to show how to mock yourtest cases.Mock yourself, not your testsexamines when mocks are necessary and when they are not as usefulso you can avoid them in your test cases.
Better tests for Redis integrations with redisliteis a great example of how using the right mocking library can cleanup existing hacky testing code and make it more straightforward forany developer that happens upon the tests in the future.
What's next after testing your application?
Can I automate testing and deployments for my app?
I want to learn more about app users via web analytics.
See also
In general, pytest is invoked with the command pytest
(see below for other ways to invoke pytest). This will execute all tests in all files whose names follow the form test_*.py
or *_test.py
in the current directory and its subdirectories. More generally, pytest follows standard test discovery rules.
Specifying which tests to run¶
Pytest supports several ways to run and select tests from the command-line.
Run tests in a module
Run tests in a directory
Run tests by keyword expressions
This will run tests which contain names that match the given string expression (case-insensitive),which can include Python operators that use filenames, class names and function names as variables.The example above will run TestMyClass.test_something
but not TestMyClass.test_method_simple
.
Run tests by node ids
Each collected test is assigned a unique nodeid
which consist of the module filename followedby specifiers like class names, function names and parameters from parametrization, separated by ::
characters.
To run a specific test within a module:
Another example specifying a test method in the command line:
Run tests by marker expressions
Will run all tests which are decorated with the @pytest.mark.slow
decorator.
For more information see marks.
Run tests from packages
This will import pkg.testing
and use its filesystem location to find and run tests from.
Getting help on version, option names, environment variables¶
Profiling test execution duration¶
To get a list of the slowest 10 test durations over 1.0s long:
By default, pytest will not show test durations that are too small (<0.005s) unless -vv
is passed on the command-line.
Managing loading of plugins¶
Early loading plugins¶
You can early-load plugins (internal and external) explicitly in the command-line with the -p
option:
The option receives a name
parameter, which can be:
A full module dotted name, for example
myproject.plugins
. This dotted name must be importable.The entry-point name of a plugin. This is the name passed to
setuptools
when the plugin isregistered. For example to early-load the pytest-cov plugin you can use:
Disabling plugins¶
To disable loading specific plugins at invocation time, use the -p
optiontogether with the prefix no:
.
Example: to disable loading the plugin doctest
, which is responsible forexecuting doctest tests from text files, invoke pytest like this:
Other ways of calling pytest¶
Calling pytest through python-mpytest
¶
You can invoke testing through the Python interpreter from the command line:
This is almost equivalent to invoking the command line script pytest[...]
directly, except that calling via python
will also add the current directory to sys.path
.
Calling pytest from Python code¶
You can invoke pytest
from Python code directly:
this acts as if you would call “pytest” from the command line.It will not raise SystemExit
but return the exit code instead.You can pass in options and arguments:
You can specify additional plugins to pytest.main
:
Running it will show that MyPlugin
was added and itshook was invoked:
Note
Html Test Runner Python Free
Calling pytest.main()
will result in importing your tests and any modulesthat they import. Due to the caching mechanism of python’s import system,making subsequent calls to pytest.main()
from the same process will notreflect changes to those files between the calls. For this reason, makingmultiple calls to pytest.main()
from the same process (in order to re-runtests, for example) is not recommended.